How to Set Up an Express Server
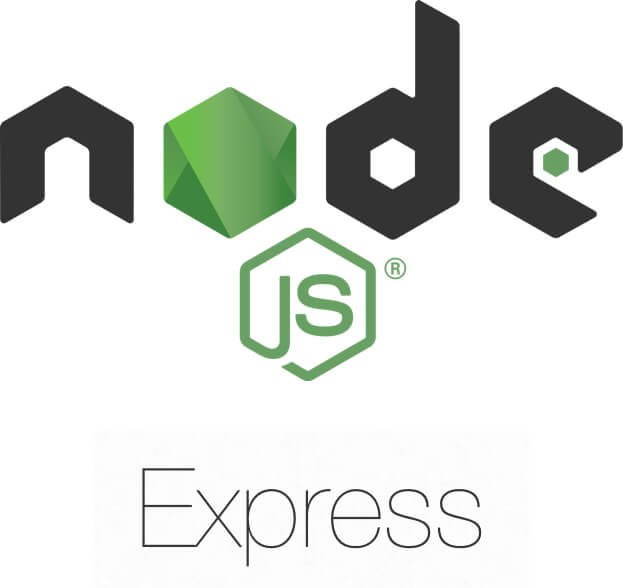
Set up an express server and nodemon.
Initial Express server setup
Learn how to set up an Express server with ease.
The first thing you need to do is make sure you're in the proper directory on your computer where you want to develop.
Make sure to create a folder for your Express server...name it whatever you want.
Cd into that directory and you can get started.
npm init
npm init -y
This creates a package.json file for us, and accepts the default settings, so we can get started setting up our application.
Install Express
npm install express --save
This will install the Express npm package for us and save it to our package.json file.
Set up index.js file
If you take a look at your package.json file, you'll notice there is a key value pair with "main": "index.js" inside.
When we used the "npm init -y" command, index.js file is set as the default file to be used for our application. This is why we will be editing index.js moving forward.
Add Express boilerplate
// Create a variable named express and set it to the return value of the require value (i.e. express)
const express = require('express');
// Execute the function stored in the express variable and set it equal to the app variable
const app = express();
// Set a port number for the app to run on
const port = 3000;
// This create a default route for our express server. The route will be http://localhost:3000
app.get('/', (req, res) => {
// Send back a response when our browser loads
http://localhost:3000
res.send('Our express app is up and running!');
})
// Start the server and listen on the port we declared above and console log that the app is running
app.listen(port, () => {
console.log(`App listening on port: http://localhost:${port}`);
})
I have added comments to the code above to help explain why it is set up the way it is. Once you have this code in your index.js file, save it, and start the app by using:
node index.js
This will start our Express server and you should see the console log in your terminal.

You can also now open your browser to http://localhost:3000 and see that the server is up and running.
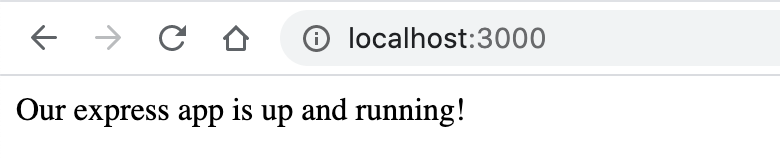
Congratulations! You have your Express server up and running and can start developing from here!