How to truncate the middle of a string
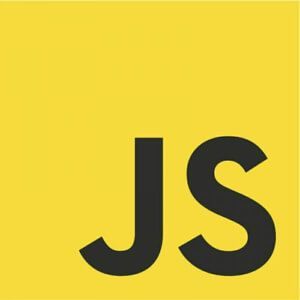
Have you ever needed to truncate a string for a mobile view?
I was recently working on a feature that required shortening a string but keeping the beginning and end of the text instead of placing the ellipsis at the end.
You're probably used to seeing something like this: I'm too long of a string, and I ...
, where you have an ellipsis at the end.
I needed mine to look like I'm too long ... and I
- since I was working with a filename and wanted to keep the extension.
This way, a person could tell what the filename was and what type of file it was. I looked around and couldn't find any CSS-only options, which meant I had to introduce some JavaScript to make this work.
Truncating in the middle of a string
Original: This is a long filename that has an extension.pdf
Needed: This is a long filen….pdf
const originalFilename = "This is a long filename that has an extension.pdf";
The first step was to split the original string to separate the filename from its extension. I used the split() method to break the string at each period, joined everything except the last part to handle multiple periods, and used pop() to retrieve the extension.
const filenameWithoutExtension = originalFilename
.split(".")
.slice(0, -1)
.join(".");
const extension = originalFilename.split(".").pop();
This code gives separate access to both the filename and the extension.
Method to format the string
Next, I created a function to format the filename:
const truncateAndFormat = (string, limit) =>
string.length <= limit ? string : `${string.slice(0, limit)}...`;
This function truncates the string if it exceeds the character limit, adds ellipses, and appends the extension.
Finally, I applied the function to format the final text:
const formattedText = `${truncateAndFormat(filenameWithoutExtension, 20)}.${extension}`;
The final solution
Now, putting this all together.
const originalFilename = "This is a long filename that has an extension.pdf";
const filenameWithoutExtension = originalFilename.split(".").slice(0, -1).join(".");
const extension = originalFilename.split(".").pop();
const truncateAndFormat = (string, limit) =>
string.length <= limit ? string : `${string.slice(0, limit)}...`;
const formattedText = `${truncateAndFormat(filenameWithoutExtension, 20)}.${extension}`;
Your formattedText
should now look like This is a long filen….pdf
.
You can also adjust the string length and where you get the ellipsis by changing the limit you pass through.