How to use React useState and useEffect hooks
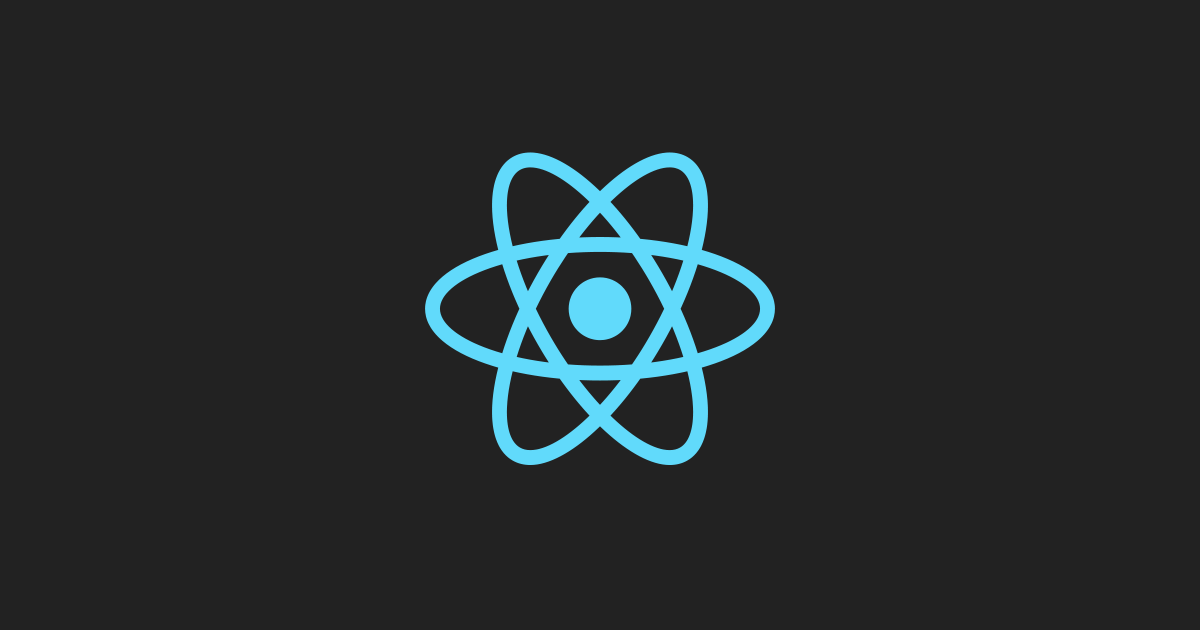
Quick overview of React's useState and useEffect hooks.
Hooks were introduced in React 16.8, and I've really enjoyed using them so far. useState is a streamlined way of working with state, and useEffect allows for us to perform side effects in function components. This way, we don't have to worry about setting up class based components and some of the boilerplate that goes along with that.
Create a sample create-react-app application
Make sure you switch into the directory where you want your application to live before starting.
cd /path/to/directory
Next we'll use the create-react-app cli to create a new react application. Name it whatever you like
npx create-react-app your-app-name-here
Once that is installed, make sure to cd into that directory, then run
npm start
This will start your application and you should see the spinning react logo.
Import useEffect and useState
Let's edit the App.js file and remove all of the boilerplate. Meaning let's cut out everything between the header tag
import React, { useState, useEffect } from 'react';
import './App.css';
function App() {
return (
<div className="App">
<h1>Hello from React</h1>
</div>
);
}
export default App;
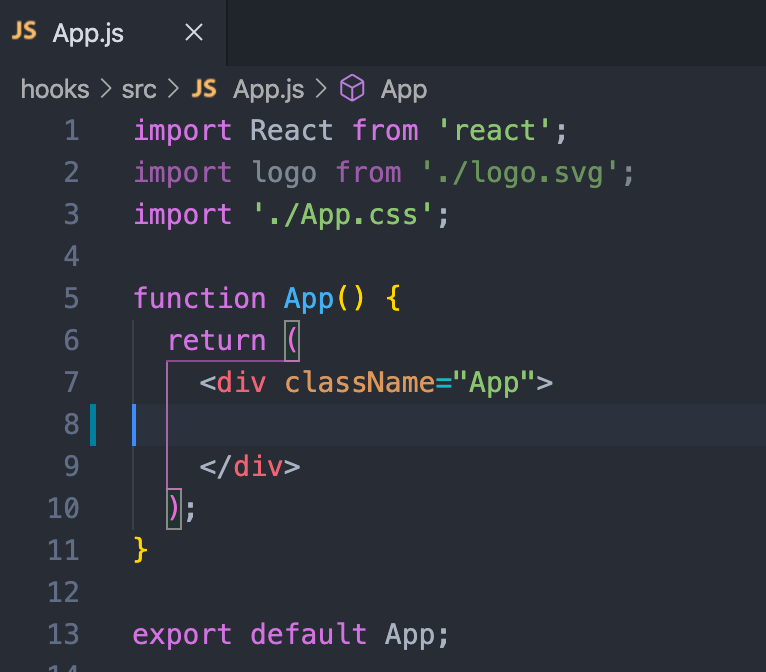
Setting up a useState hook
We will use array destructuring syntax to declare both the name of the variable we want to use, as well as the name of the function we'll use to update the state variable.
function App() {
const [count, setCount] = useState(12);
return (
<div className="App">
<h1>Hello from React</h1>
<p>Our count is: {count}</p>
</div>
);
}
Count is the variable we will use to hold the state, and setCount is the function we'll call to update the count variable.
Our app should now load like this.
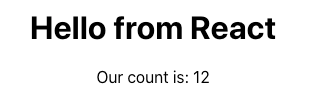
Setting up the useEffect hook
Now that we have our useState hook working, we'll add to it using the useEffect hook. useEffect provides similar functionality as componentDidMount and componentDidUpdate. We'll add it below our useState declaration.
const [count, setCount] = useState(12);
useEffect(() => {
// do something in here
});
Since we've added useEffect, we'll console.log our count variable out instead of having it load in the DOM.
function App() {
const [count, setCount] = useState(12);
useEffect(() => {
console.log(`Count is: ${count}`);
});
return (
<div className="App">
<h1>Hello from React</h1>
</div>
);
}
We should now see 12 coming from our console log statement.

Let's go ahead and add a button to increment the count.
function App() {
const [count, setCount] = useState(12);
useEffect(() => {
console.log(`Count is: ${count}`);
});
return (
<div className="App">
<h1>Hello from React</h1>
<button onClick={() => setCount(count + 1)}>Count +1</button>
</div>
);
}
Try clicking the button a few times and see what we get in the console. It should increment by 1 each time you press the button.