JavaScript's filter method
Learn how to work with JavaScript's filter method. A great way to specify the information you want to use from an array.
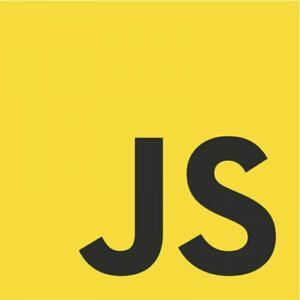
What does filter do?
The filter method creates a new array from the values that pass the test of the function you provide it.
Say you want to return all items that don't equal a certain value. This is a great use case for using the filter method.
If you have an array full of numbers and you want an array that only contains even numbers, you can use filter.
const nameArray = [2, 5, 124, 819, 4, 20, 18];
const nameArray = [2, 5, 124, 819, 4, 20, 18];
const evenNumbers = nameArray.filter(number => number % 2 === 0);
console.log(evenNumbers);
You'll see that the values that get logged out are our even numbers.

You could also reverse this and return only the odd numbers.
const nameArray = [2, 5, 124, 819, 4, 20, 18];
const oddNumbers = nameArray.filter(number => number % 2 !== 0);
console.log(oddNumbers);

You can run any sort of function to filter out elements that either equal, or don't equal a specific value. It makes it really clean to be able to get exactly what you want out of an array.
Other use cases
- Filter a name from an array of names
- Filter out undefined or NaN
- Filter out other erroneous values from an array
- Remove keys from a mapped over list in a dropdown menu
- Filter repeated values from an array
- Get an array of names that have a length > 4
- Many others
These are just a few.