JavaScript's Reduce
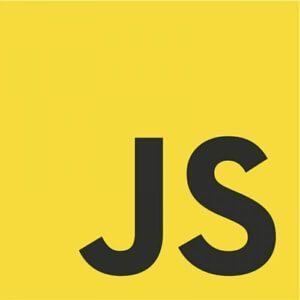
What does reduce do?
Reduce executes a function on each element in the array that you pass it. This"function" is a function you create that is also known as the reducer function.
myArray.reduce(callback( accumulator, currentValue[, index[, array]]) {
// return result from executing something on the accumulator or currentValue
}[, initialValue]);
Syntax
accumulator: It's essentially the returned value from the previous iteration of the reduce function
currentValue: The current value in the array that's being processed
index: The index of the element in the array
array: The array the reduce method was called on
The syntax seems a bit confusing when you first look at it, but it will make more sense using our example below.
Commonly used
Mathematical expressions
Simple reduce example
We're going to start with a reducer function that is going to sum all of the items in an array.
You'll generally start off with an array of items
const arrayToSum = [1, 3, 5, 23, 2, 58];
const reducerFunction = (accumulator, currentValue) => // Your reducer function logic here
What we want to do is use the accumulator to sum up the values from our array
const arrayToSum = [1, 3, 5, 23, 2, 58];
const reducerFunction = (accumulator, currentValue) =>
accumulator + currentValue;
Since we're using ES6, our reducerFunction automatically returns the value from accumulator + currentValue.
Now that our reducerFunction is created, we can test it out.
const arrayToSum = [1, 3, 5, 23, 2, 58];
const reducerFunction = (accumulator, currentValue) =>
accumulator + currentValue;
console.log(arrayToSum.reduce(reducerFunction)); // Returns 92
In this example:
- Our reducer function accepts two parameters, the accumulator and the currentValue
- Our reducerFunction then goes through each item in arrayToSum
- On each iteration, the currentValue in the array is added to our "accumulator" (which is essentially the sum)
- Finally - once the reducerFunction has gone through each of the items in the array, it returns the value stored in the accumulator
You can almost think of it as using a sum variable in a for loop. We loop overeach item in the array and add to the sum.
What if we want to start off with a different initialValue?
Well, we can add it as a second parameter to reduce like so:
const arrayToSum = [1, 3, 5, 23, 2, 58];
const reducerFunction = (accumulator, currentValue) =>
accumulator + currentValue;
console.log(arrayToSum.reduce(reducerFunction, 5)); // Returns 97