Mock Axios in Jest
You don't actually want to run Axios API calls in your tests. Here's how you mock Axios in Jest.
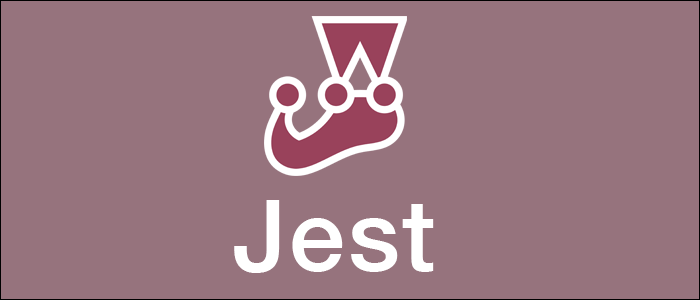
If you need to mock axios while running tests with jest, it only requires three steps.
- Import axios into your test file
- Mock the axios library
- Spy on individual axios methods to return whatever you need
import axios from "axios";
jest.mock("axios");
jest.spyOn(axios, 'get').mockResolvedValue({
data: "I'm some sample data"
});
You can also spy on any of the other axios methods (put/post/etc.) and return whatever values you need.
Now whenever you're testing a method that has an axios get request in it, your test will return "data: I'm some sample data" instead of running the actual get call from axios.