Saving work in progress while switching between branches in git
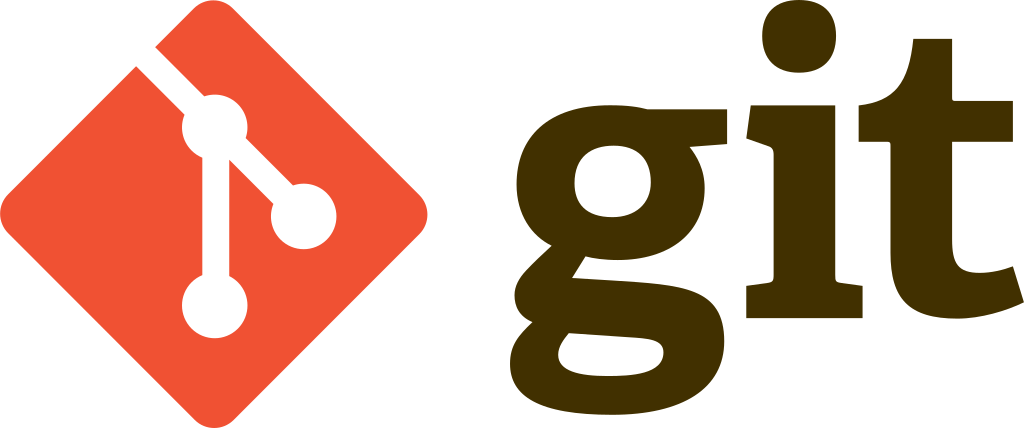
Learn how to save WIP and switch to another branch using a "temporary" commit
Imagine you’re working on a layout for a blog post on a branch called branch-1. While debugging and refining your changes, you realize there’s a high-priority bugfix that needs to be addressed on another branch. But how do you save your work-in-progress (WIP) on branch-1 so you can switch over to the bugfix branch without losing your progress?
One common approach is to use git stash, which temporarily stores your modifications, allowing you to switch contexts without committing incomplete work. This method is ideal for quick changes or when you want to keep your commit history clean during experimentation. However, git stash can sometimes lack transparency—especially if you’re juggling multiple stashes or working on longer-term tasks. In such cases, creating a temporary WIP commit offers a structured and clear alternative, ensuring your changes are explicitly saved and visible in your Git history. This article focuses on the WIP commit approach and explores when it might be a better fit for your workflow.
Overview of saving work in progress
- Make whatever changes you need
- Add a commit (prefix it with something like "WIP:")
- Move over to your source branch, create a new feature branch off of that
- Make whatever changes you need and commit on your second feature/bugfix branch
- When you're ready, switch back to your original WIP branch and add whatever changes are needed and add to your first commit using the `--amend` option. This will add your new changes to your original commit and have it in one nice commit
First set of changes
Recently I've been using temporary git commits as a way to save my WIP while switching to another branch. It's acted as a very clean and efficient way of being able to switch between features/branches without losing any of my current work, and not muddying up my commit history either. Let's look at a simple change I have in file1.js.
console.log("file 1");
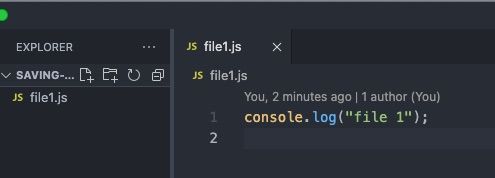
Now I realize I need to switch to another branch and work on a feature/bug real quick. What you can do in this instance is make a commit with these changes that we'll really only use as a temporary commit.
Temporarily save your changes using a commit
git status // Check the status to make sure you add the right file
git add file1.js // Stage the file
git commit -m "WIP: Whatever message you like here"
git status // Should show a clean branch
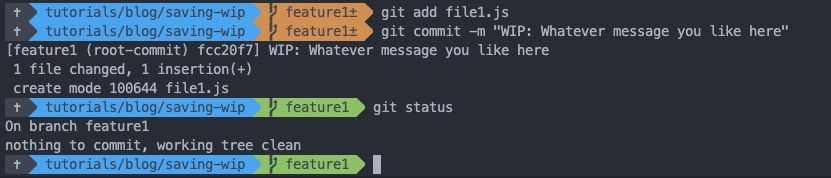

This WIP has been "saved" as a temporary git commit, allowing me to switch to another branch/feature without worrying about losing my incomplete code.
git status also shows that we have a clean working tree.
Switch to bugfix branch
You can then switch over to your bugfix branch without worrying about losing any of your changes. Switch back to your primary/main branch
git checkout main // Go back to your primary branch
git pull // Get the most recent updates
git checkout -b bugfix // Switch to new bugfix branch you want to work on
git status
Make changes in bugfix branch
Make some changes in whatever file you need to make changes in (this example will be file2.js).
console.log("bugfix changes");
Commit your changes to your bugfix branch
Once you've finished your changes to your bugfix branch, commit your changes, then go back to your feature1 branch.
git status // Check your changes
git add file2.js // Stage your changes
git commit -m "Bugfix changes" // Commit the file
git status // Check that things are clean
Switch back to your feature1 branch that has unfinished work
git checkout feature1
git status
This should be showing a clean working tree. Check git log to confirm your WIP commit is still there.

Finish changes and add to your WIP commit without creating a new commit
Let's add another change to this WIP commit to complete our changes.
console.log("file 1");
console.log("second set of changes");
git status
git add file1.js
What you can actually do is add your second set of changes to your first commit (and not create a second commit) by using --amend.
git commit -m "Final changes for feature1" --amend


You'll see that the second set of changes was added to your first commit.
You can then continue working however you like.
What if I want bring back the first commit into a "working state" instead of having them committed?
You can do a soft reset using git reset HEAD~1 to bring all the changes from that first commit back into a "Changes to be committed" state where you can make edits. Then you can commit all the changes however you like.